https://cdn.jsdelivr.net/gh/quicktouch/image@main/img
思路
最近需要实现这么一个效果:
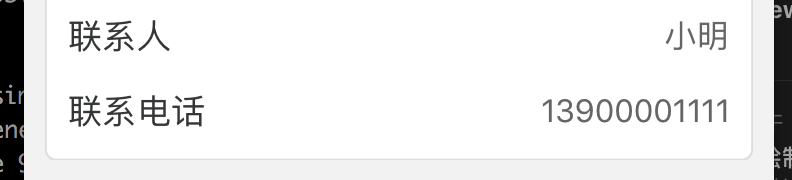
1). 因为涉及到多种类型cell、以及cell左右边距可以灵活修改,可以创建baseCell
作为父类,绘制的操作在父类统一完成。
2). 子类添加约束的时候勾选上: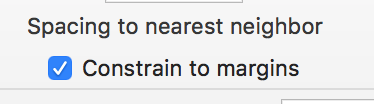
3). 在父类可以统一修改magins
,核心代码:
- (void)sg_viewLayout{
CGFloat top = self.contentView.layoutMargins.top;
CGFloat bottom = self.contentView.layoutMargins.bottom;
self.contentView.layoutMargins = UIEdgeInsetsMake(top, self.leftOrRightMagin + 2,
bottom, self.leftOrRightMagin + 2);
[self layoutIfNeeded];
[self setNeedsDisplay];
}
实现代码举例
@interface SGOrederBaseTableViewCell : UITableViewCell
@property(nonatomic,assign)BOOL isTopCell;
@property(nonatomic,assign)BOOL isBottomCell;
@property(nonatomic,assign)BOOL isMiddleCell;
@property(nonatomic,assign)BOOL isSingleCell;
@property(nonatomic,assign)CGFloat cornerRadii;
@property(nonatomic,assign)CGFloat leftOrRightMagin;
@end
@implementation SGOrederBaseTableViewCell
- (void)setIsTopCell:(BOOL)isTopCell{
[self resetCellType];
_isTopCell = isTopCell;
[self sg_viewLayout];
}
- (void)setIsBottomCell:(BOOL)isBottomCell{
[self resetCellType];
_isBottomCell = isBottomCell;
[self sg_viewLayout];
}
- (void)setIsMiddleCell:(BOOL)isMiddleCell{
[self resetCellType];
_isMiddleCell = isMiddleCell;
[self sg_viewLayout];
}
- (void)setIsSingleCell:(BOOL)isSingleCell{
[self resetCellType];
_isSingleCell = isSingleCell;
[self sg_viewLayout];
}
- (void)resetCellType{
_isSingleCell = NO;
_isMiddleCell = NO;
_isBottomCell = NO;
_isTopCell = NO;
}
- (void)sg_viewLayout{
CGFloat top = self.contentView.layoutMargins.top;
CGFloat bottom = self.contentView.layoutMargins.bottom;
self.contentView.layoutMargins = UIEdgeInsetsMake(top, self.leftOrRightMagin + 2,
bottom, self.leftOrRightMagin + 2);
[self layoutIfNeeded];
}
- (void)awakeFromNib {
[super awakeFromNib];
[self commonInit];
}
- (instancetype)initWithFrame:(CGRect)frame
{
self = [super initWithFrame:frame];
if (self) {
[self commonInit];
}
return self;
}
- (void)commonInit{
self.selectionStyle = UITableViewCellSelectionStyleNone;
self.backgroundColor = [UIColor sg_colorWithHex:0xf5f5f5];
_cornerRadii = 5.0f;
_leftOrRightMagin = 10.0f;
}
- (void)layoutMarginsDidChange{
[super layoutMarginsDidChange];
}
- (void)drawRect:(CGRect)rect{
[super drawRect:rect];
UIColor *strokeColor = [UIColor colorWithRed:211/255.0f green:211/255.0f blue:211/255.0f alpha:0.5];
CGPoint topleft = CGPointMake(self.leftOrRightMagin + 1, 0);
CGPoint bottomLeft = CGPointMake(self.leftOrRightMagin + 1, rect.size.height);
CGPoint topRight = CGPointMake(rect.size.width-1 - self.leftOrRightMagin, 0);
CGPoint bottomRight = CGPointMake(rect.size.width-1 - self.leftOrRightMagin, rect.size.height);
[strokeColor setStroke];
[[UIColor whiteColor] setFill];
CGContextRef context = UIGraphicsGetCurrentContext();
CGRect circleRect = CGRectMake(self.leftOrRightMagin + 1, 0, rect.size.width-2 - self.leftOrRightMagin * 2, rect.size.height-1);
UIBezierPath *path = nil ;
if (_isSingleCell) {
[strokeColor setStroke];
[[UIColor whiteColor] setFill];
path = [UIBezierPath bezierPathWithRoundedRect:circleRect
byRoundingCorners:UIRectCornerAllCorners
cornerRadii:CGSizeMake(self.cornerRadii, self.cornerRadii)];
[path fill];
[path stroke];
return;
}
if (_isTopCell) {
CGRect rect2 = circleRect ;
rect2.size.height = rect2.size.height + 1 ;
path = [UIBezierPath bezierPathWithRoundedRect:rect2
byRoundingCorners:UIRectCornerTopLeft| UIRectCornerTopRight
cornerRadii:CGSizeMake(self.cornerRadii, self.cornerRadii)];
[path fill];
[path stroke];
return;
}
if (_isMiddleCell) {
CGContextFillRect(context, CGRectMake(self.leftOrRightMagin + 1, 0, rect.size.width-2 - self.leftOrRightMagin * 2, rect.size.height));
[self drawLineFrom:topleft to:bottomLeft];
[self drawLineFrom:topRight to:bottomRight];
strokeColor = [UIColor blackColor];
[self drawLineFrom:CGPointMake(self.leftOrRightMagin, bottomRight.y) to:bottomRight];
return;
}
if (_isBottomCell) {
path = [UIBezierPath bezierPathWithRoundedRect:circleRect
byRoundingCorners:UIRectCornerBottomLeft | UIRectCornerBottomRight
cornerRadii:CGSizeMake(self.cornerRadii, self.cornerRadii)];
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextAddPath(context, path.CGPath);
CGContextFillPath(context);
[self drawLineFrom:topleft to:CGPointMake(bottomLeft.x, bottomLeft.y-self.cornerRadii)];
[self drawLineFrom:topRight to:CGPointMake(bottomRight.x, bottomLeft.y-self.cornerRadii)];
[self drawLineFrom:CGPointMake(bottomLeft.x+self.cornerRadii, bottomLeft.y) to:CGPointMake(bottomRight.x-self.cornerRadii, bottomRight.y)];
CGPoint centerLeft = CGPointMake(bottomLeft.x+self.cornerRadii, bottomLeft.y-self.cornerRadii) ;
CGPoint centerRight = CGPointMake(bottomRight.x-self.cornerRadii, bottomLeft.y-self.cornerRadii) ;
CGContextAddArc(context, centerLeft.x, centerLeft.y, self.cornerRadii, M_PI/2, M_PI, NO);
CGContextStrokePath(context);
CGContextAddArc(context, centerRight.x, centerRight.y, self.cornerRadii, 0, M_PI/2, NO);
CGContextStrokePath(context);
return;
}
}
@end
//ps
-(void)drawLineFrom:(CGPoint)startPoint
to:(CGPoint)endPoint
{
CGContextRef context = UIGraphicsGetCurrentContext();
CGContextMoveToPoint(context, startPoint.x, startPoint.y);
CGContextAddLineToPoint(context, endPoint.x,endPoint.y);
CGContextStrokePath(context);
}